Today the discussion is about Object Oriented Programming in Java.
In real life, an object is any entity that can be identify. The following are all objects:

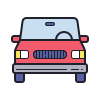

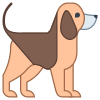

So how do you identify an object?
You identify a Person object by using the name of the Person object.
You identify a Car object by using the model or registration number of the Car object
These object identifications are represented as variables of an object in Java.
So how do you begin to create an object in Java?
You begin creating objects in Java by creating a class for each object you desire in your application.
A class represent a template or a factory from which an object is created.
For an example, a Person class represent a template from which a Person object is created.
So How do you create a class that can be used to create an object?
You create a class that can be used to create an object as follows:
public class Person {
}
Note that a class name starts with a capital letter and then an opening curly bracket:And then a closing curly bracket.
So what do you think is missing in the Person class?
Yes, the identification variables for a Person object are missing. So include a name variable of type String and what you now have is this:
public class Person {
String name;
}
The Person class is of no use without a Person constructor. A Person constructor serves as the tool or machinery used in the factory called Person class to create Person objects:
public class Person {
String name;
public Person(String name) {
this.name = name;
}
}
The Person constructor is a method that takes a name variable of type string from user input. The name input by the user is then assigned to the object by calling “this” on the name variable of the Person class.
After creating an object, how do you retrieve information about the object?
That is why you need another method in the Person class to get the name information of the object:
public class Person {
String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
You can now create a person named Donald Trump in a main method by using the Person class :
public class Main {
public static void main(String[] args) {
/**create a Person object called Donald Trump, by calling the Person constructor using new keyword*/
Person donald = new Person("Donald Trump");
/** get name of Person object called Donald*/
System.out.println(donald.getName());
}
}
For a more detailed information about object-oriented programming in Java go to
https://www.amazon.com/dp/B0DG45KX7D?ref_=pe_93986420_774957520
The code for this tutorial can be downloaded at:
https://github.com/tundetubo/Tutorials/tree/8383363337fef20a058e32ac4e312678467445a5